[파이썬 프로그래밍] GIF 제작 코딩
요약
- 파이썬 코드를 이용해 GIF 이미지를 생성하는 방법에 대해 설명합니다.
- pillow와 imageio 라이브러리를 사용하여 이미지를 처리하고 GIF로 저장합니다.
- 지정된 디렉토리에서 이미지 파일을 읽어 순차적으로 프레임을 생성하여 최종적으로 GIF 파일을 저장합니다.
간단하게 gif 를 만드는 파이썬 코드를 실습해보겠습니다.
pip install pillow imageio
from PIL import Image
import numpy as np
import imageio
import os
# Load the image
img_path = "/Users/soundfury37gmail.com/Downloads/animation_experiment/image.png" # Adjust this path to your image location
image = Image.open(img_path)
# Convert the image to an array
img_array = np.array(image)
# Create a list to store frames
frames = []
# Generate frames for the animation
for i in range(60):
shift = i % img_array.shape[1]
new_img = np.roll(img_array, shift, axis=1)
frames.append(new_img)
# Convert frames back to images
frames = [Image.fromarray(frame) for frame in frames]
# Save the frames as a GIF in the same folder as the source image
gif_path = os.path.join(os.path.dirname(img_path), "animated_image.gif")
imageio.mimsave(gif_path, frames, duration=0.1)
print(f"GIF saved at {gif_path}")
다음은 주어진 코드의 설명과 함께 단계별로 정리한 코드입니다. 이 코드는 지정된 디렉토리에서 이미지를 로드하고, 이를 애니메이션 GIF로 저장합니다.
# 필요한 라이브러리 설치
pip install pillow imageio
# 라이브러리 임포트
from PIL import Image
import imageio
import os
# 이미지가 저장된 디렉토리 경로
dir_path = "/Users/soundfury37gmail.com/Downloads/animation_experiment/"
# 디렉토리 내의 모든 이미지 파일 목록 가져오기
image_files = [f for f in os.listdir(dir_path) if os.path.isfile(os.path.join(dir_path, f)) and f.lower().endswith(('.png', '.jpg', '.jpeg'))]
# 이미지를 프레임으로 로드하여 리스트에 저장
frames = []
for image_file in image_files:
img_path = os.path.join(dir_path, image_file)
image = Image.open(img_path)
frames.append(image)
# 프레임을 GIF 파일로 저장
gif_path = os.path.join(dir_path, "animated_images.gif")
imageio.mimsave(gif_path, frames, duration=1) # 필요에 따라 duration 조정 가능
print(f"GIF saved at {gif_path}")
코드 설명
필요한 라이브러리 설치
pip install pillow imageio
pillow
: 이미지 처리 라이브러리imageio
: 이미지와 비디오를 읽고 쓰기 위한 라이브러리
라이브러리 임포트
from PIL import Image import imageio import os
이미지가 저장된 디렉토리 경로 설정
dir_path = "/Users/soundfury37gmail.com/Downloads/animation_experiment/"
디렉토리 내의 모든 이미지 파일 목록 가져오기
image_files = [f for f in os.listdir(dir_path) if os.path.isfile(os.path.join(dir_path, f)) and f.lower().endswith(('.png', '.jpg', '.jpeg'))]
os.listdir(dir_path)
: 디렉토리 내의 모든 파일과 디렉토리를 리스트로 반환os.path.isfile()
: 파일인지 확인f.lower().endswith()
: 파일 확장자가.png
,.jpg
,.jpeg
인지 확인
이미지를 프레임으로 로드하여 리스트에 저장
frames = [] for image_file in image_files: img_path = os.path.join(dir_path, image_file) image = Image.open(img_path) frames.append(image)
프레임을 GIF 파일로 저장
gif_path = os.path.join(dir_path, "animated_images.gif") imageio.mimsave(gif_path, frames, duration=1) # 필요에 따라 duration 조정 가능
imageio.mimsave()
: 여러 이미지를 하나의 애니메이션 파일로 저장duration=1
: 각 프레임이 표시되는 시간을 초 단위로 설정
결과 출력
print(f"GIF saved at {gif_path}")
이 코드는 지정된 디렉토리에서 이미지를 불러와 애니메이션 GIF로 저장하는 작업을 수행합니다. duration
값을 조정하여 각 프레임의 표시 시간을 변경할 수 있습니다.
공유하기

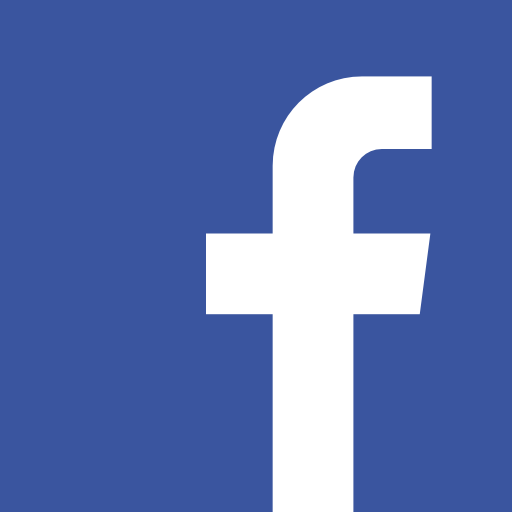

조회수 : 1180